Square in Autolayout in Swift 3/4
Sometimes I marvel about how StackOverflow has been taken over by a zealous group of idiots who close questions at will. Here's today's example, and below is my answer, since I can't put it on the SO question itself.
extension UIView {
func constrainToSquareRelativeToView(_ view: UIView, multiplier: CGFloat = 1.0) {
translatesAutoresizingMaskIntoConstraints = false
let widthConstraint = NSLayoutConstraint(item: self, attribute: .width, relatedBy: .lessThanOrEqual, toItem: view, attribute: .width, multiplier: multiplier, constant: 0)
widthConstraint.priority = .defaultLow
let heightConstraint = NSLayoutConstraint(item: self, attribute: .height, relatedBy: .equal, toItem: view, attribute: .height, multiplier: multiplier, constant: 0)
heightConstraint.priority = .defaultLow
let squareConstraint = NSLayoutConstraint(item: self, attribute: .height, relatedBy: .equal, toItem: self, attribute: .width, multiplier: 1.0, constant: 0)
let centerX = NSLayoutConstraint(item: self, attribute: .centerXWithinMargins, relatedBy: NSLayoutRelation.equal, toItem: view, attribute: .centerXWithinMargins, multiplier: 1, constant:0)
let centerY = NSLayoutConstraint(item: self, attribute: .centerYWithinMargins, relatedBy: NSLayoutRelation.equal, toItem: view, attribute: .centerYWithinMargins, multiplier: 1, constant:0)
view.addConstraints([widthConstraint, heightConstraint, squareConstraint, centerX, centerY])
}
}
Here's my result (inside which I'm playing with a UICollectionView, but that's another story):
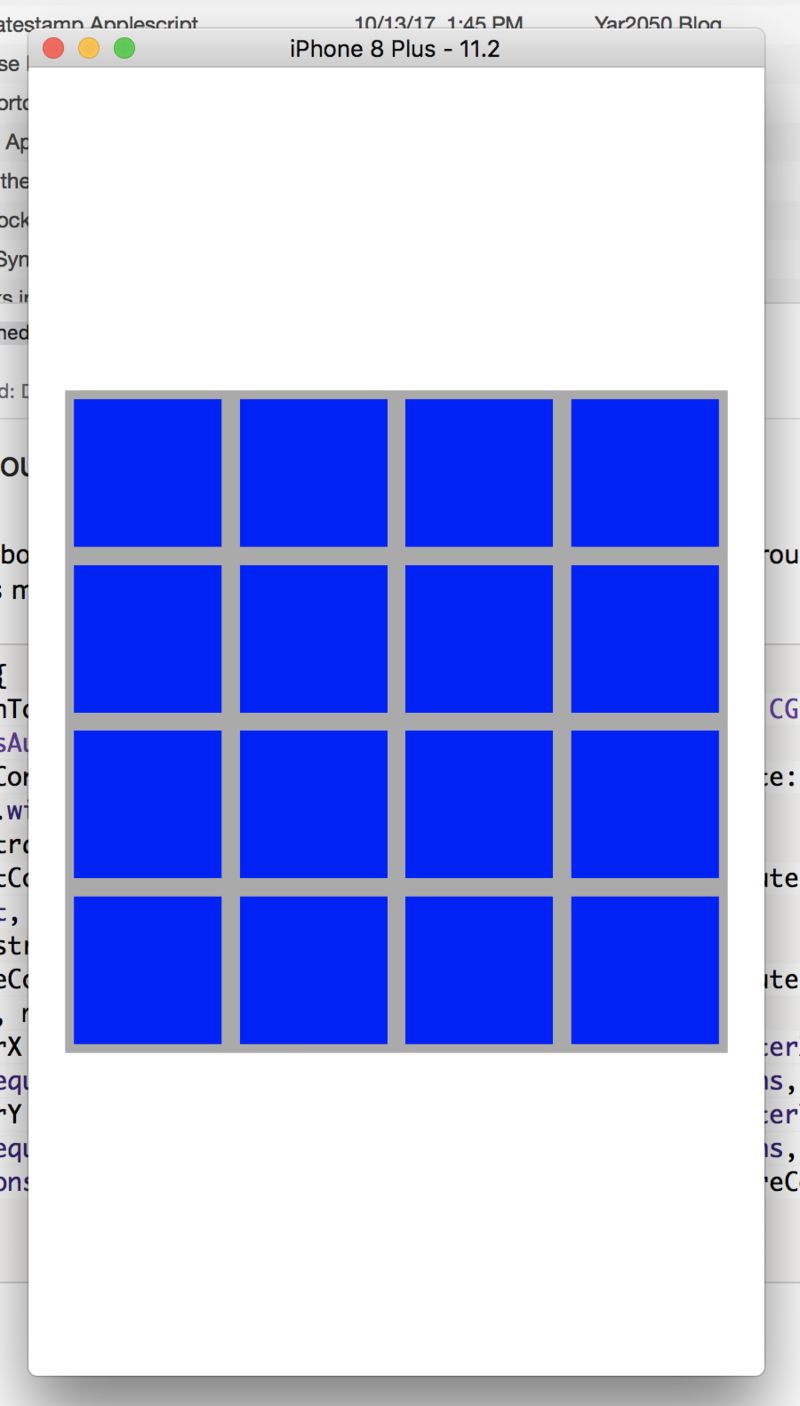