Showing all posts tagged "Swift3"
Just Because It Compiles: More Notes from Swift 3 Upgrade
This is the method signature for the superclass:
public func sendRequest<T: Request>(request: T, handler: (Result<T.Response, Error>) -> Void) -> NSURLSessionDataTask? {
and this is in the subclass
override func sendRequest<T : RequestType>(request: T, handler: (Result<T.Response, PayLib.Error>) -> Void) -> NSURLSessionDataTask? {
and in Swift 3 this got translated to be the same, except for some minor changes:
open func sendRequest<T: Request>(_ request: T, handler: @escaping (Result<T.Response, Error>) -> Void) -> URLSessionDataTask? {
Subclass:
override func sendRequest<T : RequestType>(_ request: T, handler: @escaping (Result<T.Response, PayLib.Error>) -> Void) -> URLSessionDataTask? ```
Now that was good enough for Swift 2. In Swift 3, a call to this method resulted in a crash with no interesting information.
The reason was the mismatch between RequestType and Request (RequestType is the protocol which the Request Protocol extends). So the fix was merely to have the signatures match exactly.
override func sendRequest<T : Request>(_ request: T, handler: @escaping (Result<T.Response, PayLib.Error>) -> Void) -> URLSessionDataTask? {
I'm not sure that there's a point here, except that: Just because Xcode likes it, it compiles and it works in Swift 2 doesn't mean that it won't cause a runtime crash in Swift 3. Or something like that: check everything by hand/eye!
Posted on October 5th, 2017
Optional Block Parameters and Fix-Its
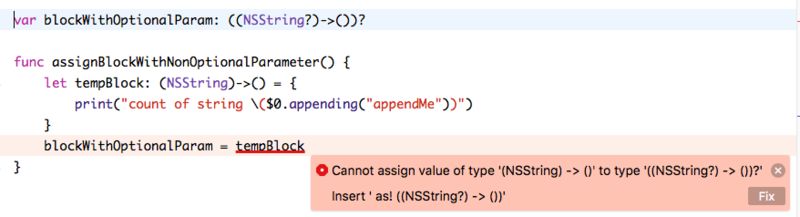
Posted on September 19th, 2017
Lazy Array in Swift
Posted on August 19th, 2017