Optional Block Parameters and Fix-Its
Consider this fix-it:
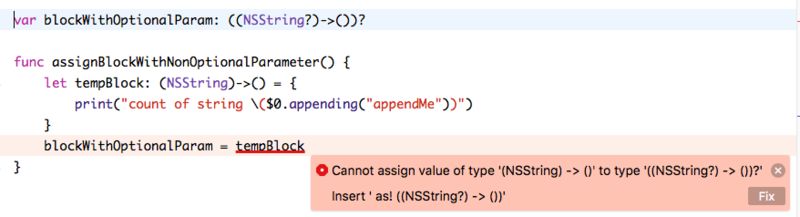
Now if this happens to you -- as it happened to me -- as part of a massive code conversion to Swift 3, you might be tempted to follow the fix it. Then your code would read like this:
var blockWithOptionalParam: ((NSString?)->())?
func assignBlockWithNonOptionalParameter() {
let tempBlock: (NSString)->() = {
print("count of string \($0.appending("appendMe"))")
}
blockWithOptionalParam = tempBlock as! ((NSString?) -> ())
}
This might be fine, but would result in a crash if you ever passed a nil to your blockWithOptionalParam. The only safe thing to do, really, is to ignore the fix it and handle the optional properly:
func assignBlockWithNonOptionalParameter() {
let tempBlock: (NSString?)->() = {
guard let text = $0 else { return }
print("count of string \(text.appending("appendMe"))")
}
blockWithOptionalParam = tempBlock
}
So What?
For some other cases -- and I'm not sure what the difference is, yet -- Xcode always suggests casting the block rather than fixing the optional in the block. I'm unable to show this example in the lab, but it does show up in the codebase I'm working on currently.