Showing all posts tagged "Xcode"
Xcodebuild: Valid Destinations on OSX?
To get a list of valid destinations, specify an erroneous key-value pair and xcodebuild will spit out the combinations that work.
List Destinations Command
xcodebuild test -destination 'platform=iOS Simulator' -workspace Register.xcworkspace -scheme ThatTestTarget
Output Example
Available destinations for the "ThatTestTarget" scheme:
{ platform:iOS Simulator, id:145A9B7E-B336-4819-8059-2FFEC408E05E, OS:11.1, name:iPad (5th generation) }
{ platform:iOS Simulator, id:69ABAF6F-ADA3-4E38-AC97-D71001447663, OS:9.3, name:iPad 2 }
{ platform:iOS Simulator, id:550E2F18-406D-4586-84BB-E48F1D704F27, OS:10.3.1, name:iPad Air }
{ platform:iOS Simulator, id:94734F1C-775F-40FA-9015-8196C08805EF, OS:11.1, name:iPad Air }
{ platform:iOS Simulator, id:1DB953DD-CD97-4EC7-8006-BCF01DF3E63F, OS:11.1, name:iPad Air 2 }
{ platform:iOS Simulator, id:DE3072DA-2E31-423D-9D77-220626F8B90A, OS:11.1, name:iPad Pro (9.7-inch) }
{ platform:iOS Simulator, id:3B5D18DB-13B5-4F28-B654-7D2ECDD1F6F0, OS:11.1, name:iPad Pro (10.5-inch) }
{ platform:iOS Simulator, id:A4225E3A-512C-4F42-ADD9-1E7E448C4D27, OS:11.1, name:iPad Pro (12.9-inch) }
{ platform:iOS Simulator, id:684FF1BA-8784-4B7C-B4E5-5231772F0FAC, OS:11.1, name:iPad Pro (12.9-inch) (2nd generation) }
Change Colons for Equals Signs, Remove Spaces, Ignore the ID
So if you want to use this destination:
platform:iOS Simulator, id:684FF1BA-8784-4B7C-B4E5-5231772F0FAC, OS:11.1, name:iPad Pro (12.9-inch) (2nd generation)
Change the colons for commas, remove the spaces, remove the ID, so you get this string:
platform=iOS Simulator,OS=11.1,name=iPad Pro (12.9-inch) (2nd generation)
Then the entire command would be:
xcodebuild test -destination 'platform=iOS Simulator,OS=11.1,name=iPad Pro (12.9-inch) (2nd generation)' -workspace Register.xcworkspace -scheme ThatTestTarget
From my StackOverflow Answer here
Posted on January 8th, 2018
Just Because It Compiles: More Notes from Swift 3 Upgrade
This is the method signature for the superclass:
public func sendRequest<T: Request>(request: T, handler: (Result<T.Response, Error>) -> Void) -> NSURLSessionDataTask? {
and this is in the subclass
override func sendRequest<T : RequestType>(request: T, handler: (Result<T.Response, PayLib.Error>) -> Void) -> NSURLSessionDataTask? {
and in Swift 3 this got translated to be the same, except for some minor changes:
open func sendRequest<T: Request>(_ request: T, handler: @escaping (Result<T.Response, Error>) -> Void) -> URLSessionDataTask? {
Subclass:
override func sendRequest<T : RequestType>(_ request: T, handler: @escaping (Result<T.Response, PayLib.Error>) -> Void) -> URLSessionDataTask? ```
Now that was good enough for Swift 2. In Swift 3, a call to this method resulted in a crash with no interesting information.
The reason was the mismatch between RequestType and Request (RequestType is the protocol which the Request Protocol extends). So the fix was merely to have the signatures match exactly.
override func sendRequest<T : Request>(_ request: T, handler: @escaping (Result<T.Response, PayLib.Error>) -> Void) -> URLSessionDataTask? {
I'm not sure that there's a point here, except that: Just because Xcode likes it, it compiles and it works in Swift 2 doesn't mean that it won't cause a runtime crash in Swift 3. Or something like that: check everything by hand/eye!
Posted on October 5th, 2017
Optional Block Parameters and Fix-Its
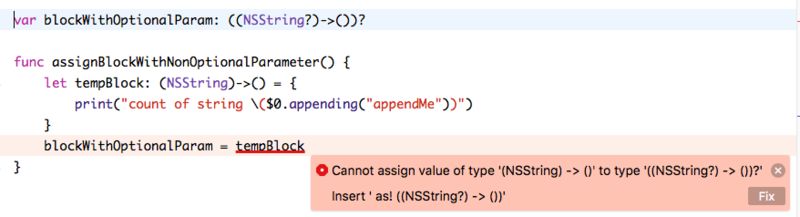
Posted on September 19th, 2017
Breakpoint Swift Deinit in Xcode
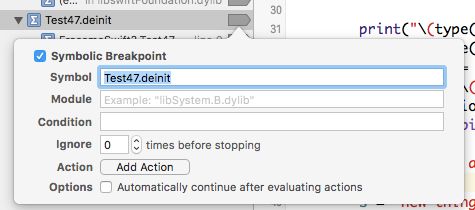
- If there is no body to your deinit method, the breakpoint will not fire (it gets optimized out or something similar).
- There is no difference in syntax, for the Xcode symbolic breakpoints, between class and instance methods.
- There are no deinit methods for structs, so your SOL on that one.
Posted on September 12th, 2017