Solution for No Optionals in Swift String Interpolation
Posted on November 6th, 2017
My Time/Datestamp Applescript
Original
Here's the .scpt file that will open in Script Editor on Mac. You can set it up as a keystroke (CMD-Shift-D for me) in FastScripts (free for up to 10 scripts).
-- yar2050 (Dan Rosenstark)'s favorite date format
-- 2017-08-03 update
on fillInZero(int)
if (int < 10) then set int to "0" & int
return int as string
end fillInZero
set theDate to (current date)
set theHour to fillInZero(hours of (theDate) as integer)
set theMinutes to fillInZero(minutes of (theDate) as integer)
tell theDate to get (its month as integer)
set theMonth to fillInZero(result)
set theDay to fillInZero(day of theDate)
tell (theDate) to get (its year as integer) & "-" & (theMonth) & "-" & theDay & " " & (theHour) & ":" & theMinutes
set date_ to (result as string)
tell application "System Events"
set frontmostApplication to name of the first process whose frontmost is true
end tell
tell application frontmostApplication
delay 0.2 -- I have no idea why this is necessary
activate
tell application "System Events"
if (frontmostApplication = "Evernote") then
keystroke "h" using {command down, shift down}
keystroke "b" using {command down}
keystroke date_
keystroke "b" using {command down}
else
keystroke date_
end if
end tell
end tell
2023-06 - Update
Working with ChatGPT we came up with this. Much better.
set formattedDate to do shell script "date '+%Y-%m-%d %H:%M'"
tell application "System Events"
keystroke formattedDate
end tell
-- display alert "AppleScript is working" message "Date: " & formattedDate
Posted on October 13th, 2017
Just Because It Compiles: More Notes from Swift 3 Upgrade
This is the method signature for the superclass:
public func sendRequest<T: Request>(request: T, handler: (Result<T.Response, Error>) -> Void) -> NSURLSessionDataTask? {
and this is in the subclass
override func sendRequest<T : RequestType>(request: T, handler: (Result<T.Response, PayLib.Error>) -> Void) -> NSURLSessionDataTask? {
and in Swift 3 this got translated to be the same, except for some minor changes:
open func sendRequest<T: Request>(_ request: T, handler: @escaping (Result<T.Response, Error>) -> Void) -> URLSessionDataTask? {
Subclass:
override func sendRequest<T : RequestType>(_ request: T, handler: @escaping (Result<T.Response, PayLib.Error>) -> Void) -> URLSessionDataTask? ```
Now that was good enough for Swift 2. In Swift 3, a call to this method resulted in a crash with no interesting information.
The reason was the mismatch between RequestType and Request (RequestType is the protocol which the Request Protocol extends). So the fix was merely to have the signatures match exactly.
override func sendRequest<T : Request>(_ request: T, handler: @escaping (Result<T.Response, PayLib.Error>) -> Void) -> URLSessionDataTask? {
I'm not sure that there's a point here, except that: Just because Xcode likes it, it compiles and it works in Swift 2 doesn't mean that it won't cause a runtime crash in Swift 3. Or something like that: check everything by hand/eye!
Posted on October 5th, 2017
Show Only Apps in Search Results, iOS 11
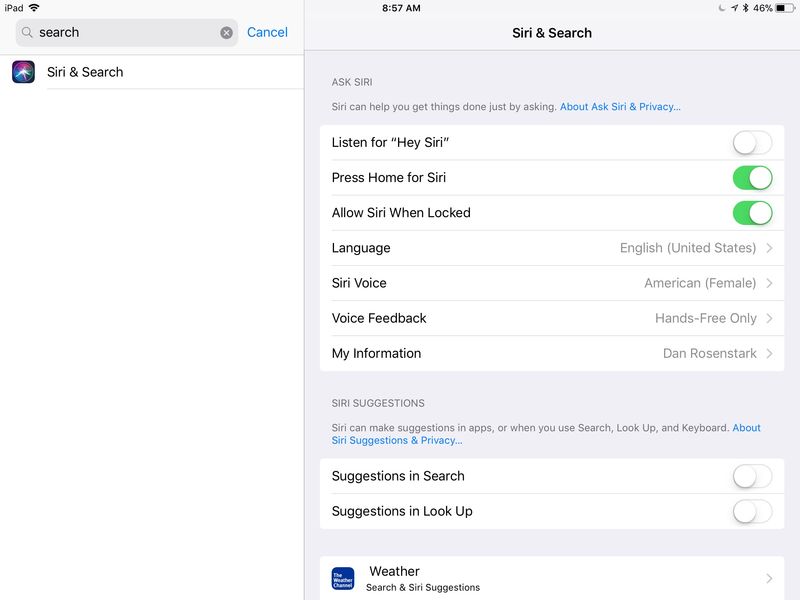
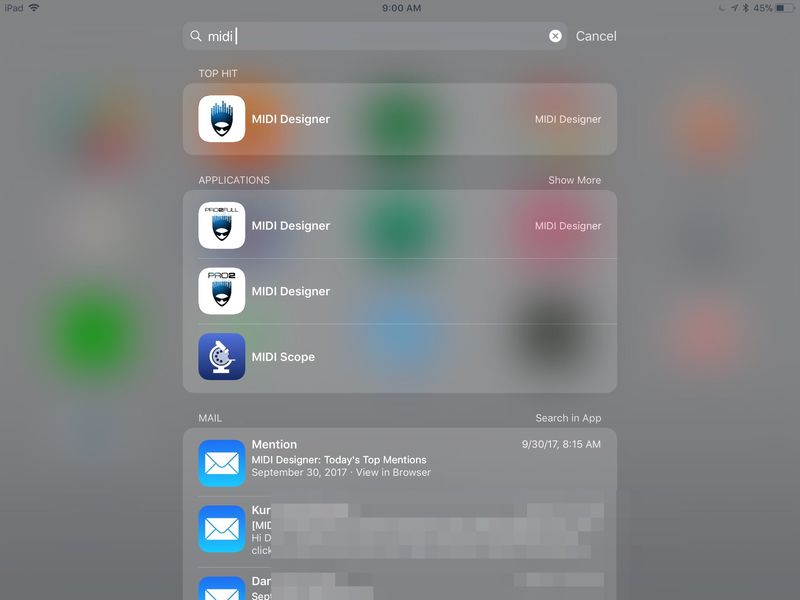
Posted on September 30th, 2017
Optional Block Parameters and Fix-Its
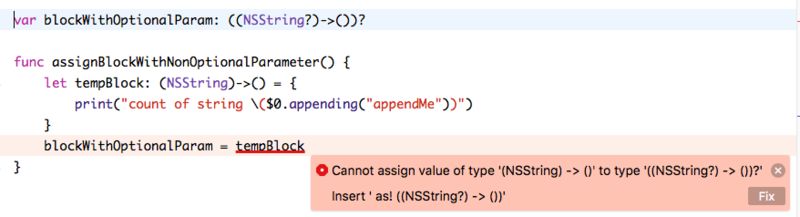
Posted on September 19th, 2017
Enabling Command-Line Pipe for Atom on OS X
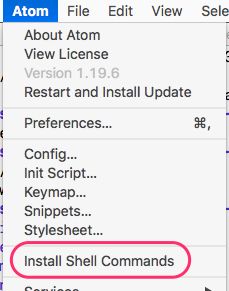
2018-02-15: Update
Posted on September 12th, 2017
Breakpoint Swift Deinit in Xcode
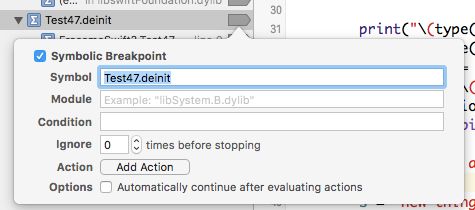
- If there is no body to your deinit method, the breakpoint will not fire (it gets optimized out or something similar).
- There is no difference in syntax, for the Xcode symbolic breakpoints, between class and instance methods.
- There are no deinit methods for structs, so your SOL on that one.
Posted on September 12th, 2017
Swift 3.x Calls Objective-C
Posted on September 8th, 2017
Swift and Object-C Interop, Simplest Possible Example
Start with a Single View App (Swift) in Xcode 9 Beta 6 called SwiftObjCInterop
.
Swift Calls Objective-C
Create an Objective-C Class
Create an Objective-C class called ObjCObject
This will automatically give you the Bridging Header to call the Objective-C from Swift (that was easy!):
Select Create Bridging Header which will produce the file SwiftObjCInterop-Bridging-Header
Add a Method to ObjCObject
Add a method like this in your .m file
- (void) test {
NSLog(@"Yes we are ObjCObject and we are testing");
}
and this in your .h
- (void) test;
Add ObjCObject to your Bridging Header
#import "ObjCObject.h"
Test Swift Calls Objective-C
Put this in your viewDidLoad
:
ObjCObject().test()
Run the project. Now Swift calls Objective-C.
Objective-C Calls Swift
This is slightly trickier in an existing project, but in a new project there are no issues.
Include this in your ViewController.swift
file:
@objc(testSwift)
func testSwift() {
print("yes ViewController is printing")
}
For some reason, you need the @objC
tag to be able to see your method from Objective-C.
Include this import in your .m file:
#import "SwiftObjCInterop-Swift.h"
And add this to your test
method in your Objective-C class:
ViewController *vc = [[ViewController alloc] init];
[vc testSwift];
That's it.
Posted on September 5th, 2017